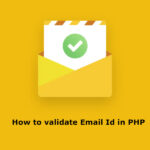
How to Do Email Validation in PHP: A Comprehensive Guide
Introduction
Email validation is an essential aspect of web development, ensuring that the email addresses provided by users are valid, correctly formatted, and meet the necessary standards. When building applications that rely on user input, such as registration forms or contact forms, it’s critical to have robust validation to avoid errors or misuse. In this article, we will guide you through the process of email validation in PHP, exploring various methods, techniques, and best practices for verifying email addresses.
Why Email Validation Is Important
Email validation plays a crucial role in safeguarding your application. If users enter incorrect or fake email addresses, it can lead to several issues, such as:
- Unsuccessful Communication: Sending emails to invalid addresses means your messages won’t reach users.
- Increased Spam Risk: Invalid or fake emails can open the door to spam or phishing attacks.
- User Experience Issues: Invalid emails might cause errors during registration, login, or password recovery processes.
Methods of Email Validation in PHP
In PHP, there are several ways to validate email addresses. Let’s go through some of the most common methods to achieve email validation in PHP.
1. Basic Email Validation Using PHP filter_var()
The easiest way to validate email addresses in PHP is by using the filter_var()
function with the FILTER_VALIDATE_EMAIL
flag. This method checks if the email format adheres to the standard format.
Here’s an example of basic email validation using filter_var()
:
This function returns true
if the email is valid, and false
if it’s not. This is a simple and quick way to validate emails.
2. Regular Expressions for Email Validation
For more control over the email validation process, you can use regular expressions (regex). Regular expressions are particularly useful if you want to implement custom email validation rules, such as validating specific domain names or patterns.
Here’s a sample regex for validating an email address in PHP:
This regular expression checks if the email matches a standard email pattern. However, be aware that regex-based email validation can become complicated if you try to account for every edge case.
3. Using PHP’s checkdnsrr()
Function
To verify that the email domain exists and is active, you can use PHP’s checkdnsrr()
function. This function checks the DNS (Domain Name System) records for the domain, ensuring that it’s a valid domain for sending emails.
This method adds an extra layer of validation by ensuring that the domain of the email address has a mail exchange (MX) record, meaning that the domain is capable of receiving emails.
4. Advanced Email Validation with Third-Party Libraries
While the methods mentioned above are useful, sometimes you may require more sophisticated email validation to handle edge cases such as domain blacklists, disposable emails, or syntactically unusual addresses. In such cases, using third-party libraries can save time and provide more accuracy.
One popular library for email validation is EmailValidator. This library implements the complete email validation standard as defined by RFC 5321 and RFC 5322, handling a wide variety of edge cases.
You can install the library via Composer:
Once installed, you can use it like this:
This method ensures that emails are thoroughly validated according to the official RFC standards.
Common Pitfalls in Email Validation
While implementing email validation in PHP, developers often encounter certain challenges. Let’s take a look at some common pitfalls and how to avoid them.
1. Over-Strict Validation
Some email validation approaches are too strict, rejecting valid but unusual email addresses. For example, an email like user@subdomain.example.com
may be valid but rejected by overly strict regular expressions. Always consider the real-world diversity of email addresses when implementing validation.
2. Ignoring Internationalized Email Addresses
With the advent of internationalized domain names (IDNs), email addresses can now contain non-ASCII characters. If your validation doesn’t account for these characters, valid email addresses might be incorrectly flagged as invalid. Make sure to account for international email addresses if your application will be used globally.
3. Failing to Check MX Records
While filter_var()
checks the format of the email address, it doesn’t confirm that the domain can actually receive emails. Using checkdnsrr()
to verify that the domain has an MX record is crucial to avoid accepting email addresses from non-existent domains.
Best Practices for Email Validation
To ensure that your email validation process is as robust as possible, consider these best practices:
- Combine Validation Methods: Use multiple validation techniques, such as format validation with
filter_var()
and domain validation withcheckdnsrr()
, for a more comprehensive approach. - Provide Real-Time Feedback: Display error messages to users immediately if their email addresses are invalid, helping them correct mistakes before submission.
- Sanitize Inputs: Even though validation checks the format of the email, always sanitize user input to avoid malicious scripts or injections.
- Consider User Experience: Don’t block valid email addresses with unusual characters or domain types. Remember to provide clear and helpful error messages.
Conclusion
Email validation in PHP is an essential part of ensuring that your web applications are secure, reliable, and user-friendly. By using the right techniques, such as the filter_var()
function, regular expressions, or even third-party libraries like EmailValidator, you can verify that email addresses meet the required standards.